
We have used many of Python’s built-in types; now we are going to define a new type. As an example, we will create a type called Point that represents a point in two-dimensional space.
In mathematical notation, points are often written in parentheses with a comma separating the coordinates. For example, (0, 0) represents the origin, and (x, y) represents the point x units to the right and y units up from the origin.
There are several ways we might represent points in Python:
- We could store the coordinates separately in two variables, x and y.
- We could store the coordinates as elements in a list or tuple.
- We could create a new type to represent points as objects.
Creating a new type is (a little) more complicated than the other options, but it has advantagesthat will be apparent soon.
A user-defined type is also called a class. A class definition looks like this:
class Point(object):"""Represents a point in 2-D space."""
This header indicates that the new class is a Point, which is a kind of object, which is a built-in type.
The body is a docstring that explains what the class is for. You can define variables and functions inside a class definition, but we will get back to that later.
Defining a class named Point creates a class object.
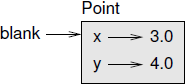
>>> print Point<class '__main__.Point'>
Because Point is defined at the top level, its “full name” is __main__.Point.
The class object is like a factory for creating objects. To create a Point, you call Point as if it were a function.
>>> blank = Point()>>> print blank<__main__.Point instance at 0xb7e9d3ac>
The return value is a reference to a Point object, which we assign to blank. Creating a new object is called instantiation, and the object is an instance of the class.
When you print an instance, Python tells you what class it belongs to and where it is stored in memory (the prefix 0x means that the following number is in hexadecimal).
- 2186 reads