
As you may have discovered, it is legal to make more than one assignment to the same variable. A new assignment makes an existing variable refer to a new value (and stop referring to the old value).
bruce = 5 print bruce, bruce = 7 print bruce
The output of this program is 57, because the first time bruce is printed, its value is 5, and the second time, its value is 7. The comma at the end of the first print statement suppresses the newline, which is why both outputs appear on the same line.
Figure 7.1 shows what multiple assignment looks like in a state diagram.
With multiple assignment it is especially important to distinguish between an assignment operation and a statement of equality. Because Python uses the equal sign (=) for assignment, it is tempting to interpret a statement like a=b as a statement of equality. It is not!
First, equality is a symmetric relation and assignment is not. For example, in mathematics, if a = 7 then 7 = a. But in Python, the statement a=7 is legal and 7=a is not.
Furthermore, in mathematics, a statement of equality is either true or false, for all time. If a = b now, then a will always equal b. In Python, an assignment statement can make two variables equal, but they don’t have to stay that way:
a = 5 b = a # a and b are now equal a = 3 # a and b are no longer equal
The third line changes the value of a but does not change the value of b, so they are no longer equal.
Although multiple assignment is frequently helpful, you should use it with caution. If the values of variables change frequently, it can make the code difficult to read and debug.
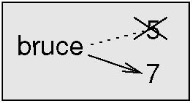
- 2137 reads